Fashion Endpoint
Overview
This endpoint allows you to wear a cloth image sample on an existing model body. The ideal input images should have white background, fully visible model body, and the cloth should be an individual piece.
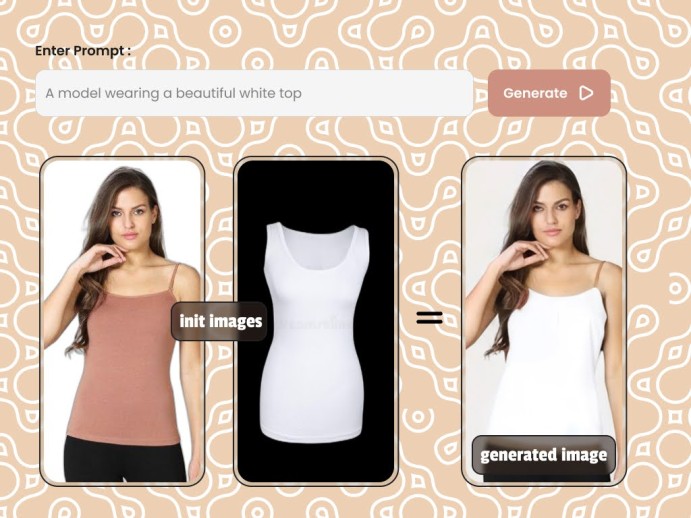
Request
--request POST 'https://modelslab.com/api/v6/image_editing/fashion' \
Make a POST
request to https://modelslab.com/api/v6/image_editing/fashion endpoint and pass the required parameters as a request body to the endpoint.
Body Attributes
Parameter | Description | Values |
---|---|---|
key | Your API Key used for request authorization | string |
init_image | The URL of the image of the model to try the dress on | URL |
cloth_image | The URL of the cloth/dress to try on | URL |
cloth_type | One of upper_body , lower_body , or dresses , based on where the garment is to be worn | upper_body , lower_body , or dresses |
prompt | The text prompt describing the things you want in the image to be generated | string |
negative_prompt | Items you do not want in the image | string |
num_inference_steps | The number of denoising steps. The acceptable values are 21, 31, and 41. | integer (21, 31, or 41) |
temp | Set to "yes" if you want proxy links to access images in addition to normal links. Useful if your country blocks the storage provider. The default is "no". | "yes" or "no" |
guidance_scale | The scale for classifier-free guidance. The minimum is 1 and the maximum is 20. | integer (1 to 20) |
webhook | Provide a URL to receive a POST API call once the image generation is complete | URL |
track_id | This ID is returned in the response to the webhook API call and will be used to identify the webhook request | integral value |
Example
Body
Body
{
"key": "",
"prompt": ":A realistic photo of a model wearing a beautiful t-shirt",
"negative_prompt": "Low quality, unrealistic, bad cloth, warped cloth",
"init_image": "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5dzoZ9qWI2FQxwceFDb3zULRtwCRmF-metaZjA5NjMyX3BhcmVudF8xXzE2NTMwMDMzODguanBn-.jpg",
"cloth_image": "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5BDmwvtizESFRO24uGDW1iu1u5TXhB-metaM2JmZmFkY2U5NDNkOGU3MDJhZDE0YTk2OTY2NjQ0NjYuanBn-.jpg",
"cloth_type": "upper_body",
"guidance_scale": 7.5,
"num_inference_steps": 21,
"seed": null,
"temp": "no",
"webhook": null,
"track_id": null
}
Request
- JS
- PHP
- NODE
- PYTHON
- JAVA
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"key": "",
"prompt": "A realistic photo of a model wearing a beautiful t-shirt",
"negative_prompt": "Low quality, unrealistic, bad cloth, warped cloth",
"init_image": "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5dzoZ9qWI2FQxwceFDb3zULRtwCRmF-metaZjA5NjMyX3BhcmVudF8xXzE2NTMwMDMzODguanBn-.jpg",
"cloth_image": "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5BDmwvtizESFRO24uGDW1iu1u5TXhB-metaM2JmZmFkY2U5NDNkOGU3MDJhZDE0YTk2OTY2NjQ0NjYuanBn-.jpg",
"cloth_type": "upper_body",
"guidance_scale": 7.5,
"num_inference_steps": 21,
"seed": null,
"temp": "no",
"webhook": null,
"track_id": null
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://modelslab.com/api/v6/image_editing/fashion", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$payload = [
"key" => "",
"prompt" => "A realistic photo of a model wearing a beautiful t-shirt",
"negative_prompt" => "Low quality, unrealistic, bad cloth, warped cloth",
"init_image" => "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5dzoZ9qWI2FQxwceFDb3zULRtwCRmF-metaZjA5NjMyX3BhcmVudF8xXzE2NTMwMDMzODguanBn-.jpg",
"cloth_image": "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5BDmwvtizESFRO24uGDW1iu1u5TXhB-metaM2JmZmFkY2U5NDNkOGU3MDJhZDE0YTk2OTY2NjQ0NjYuanBn-.jpg",
"cloth_type" => "upper_body",
"guidance_scale" => 7.5,
"num_inference_steps" => 21,
"seed" => null,
"temp" => "no",
"webhook" => null,
"track_id" => null
];
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://modelslab.com/api/v6/image_editing/fashion',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => json_encode($payload),
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://modelslab.com/api/v6/image_editing/fashion',
'headers': {
'Content-Type': 'application/json'
},
body: JSON.stringify({
"key": "",
"prompt" => "A realistic photo of a model wearing a beautiful t-shirt",
"negative_prompt": "Low quality, unrealistic, bad cloth, warped cloth",
"init_image" => "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5dzoZ9qWI2FQxwceFDb3zULRtwCRmF-metaZjA5NjMyX3BhcmVudF8xXzE2NTMwMDMzODguanBn-.jpg",
"cloth_image": "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5BDmwvtizESFRO24uGDW1iu1u5TXhB-metaM2JmZmFkY2U5NDNkOGU3MDJhZDE0YTk2OTY2NjQ0NjYuanBn-.jpg",
"cloth_type": "upper_body",
"guidance_scale": 7.5,
"num_inference_steps": 21,
"seed": null,
"temp": "no",
"webhook": null,
"track_id": null
})
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
import requests
import json
url = "https://modelslab.com/api/v6/image_editing/fashion"
payload = json.dumps({
"key": "",
"prompt" => "A realistic photo of a model wearing a beautiful t-shirt",
"negative_prompt": "Low quality, unrealistic, bad cloth, warped cloth",
"init_image" => "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5dzoZ9qWI2FQxwceFDb3zULRtwCRmF-metaZjA5NjMyX3BhcmVudF8xXzE2NTMwMDMzODguanBn-.jpg",
"cloth_image": "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5BDmwvtizESFRO24uGDW1iu1u5TXhB-metaM2JmZmFkY2U5NDNkOGU3MDJhZDE0YTk2OTY2NjQ0NjYuanBn-.jpg",
"cloth_type": "upper_body",
"guidance_scale": 7.5,
"num_inference_steps": 21,
"seed": null,
"temp": "no",
"webhook": None,
"track_id": None
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n \"key\": \"\",\n \"prompt\": \"A realistic photo of a model wearing a beautiful t-shirt\",\n \"negative_prompt\": \"Low quality, unrealistic, bad cloth, warped cloth\",\n \"init_image\":https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5dzoZ9qWI2FQxwceFDb3zULRtwCRmF-metaZjA5NjMyX3BhcmVudF8xXzE2NTMwMDMzODguanBn-.jpg\"\",\n \"cloth_image\": \"https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5BDmwvtizESFRO24uGDW1iu1u5TXhB-metaM2JmZmFkY2U5NDNkOGU3MDJhZDE0YTk2OTY2NjQ0NjYuanBn-.jpg\",\n \"cloth_type\": \"upper_body\",\n \"guidance_scale\": 7.5,\n \"num_inference_steps\": 21,\n \"seed\": null,\n \"temp\": \"no\",\n \"webhook\": null,\n \"track_id\": null \n}");
Request request = new Request.Builder()
.url("https://modelslab.com/api/v6/image_editing/fashion")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.build();
Response response = client.newCall(request).execute();
Response
Example Response
{
"status": "success",
"generationTime": 4.713963508605957,
"id": 103204,
"output": [],
"proxy_links": [
"https://cdn2.stablediffusionapi.com/generations/284c4f3d-4bf6-4bad-a603-d2a1948de0cf.png"
],
"meta": {
"base64": "no",
"cloth": "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5BDmwvtizESFRO24uGDW1iu1u5TXhB-metaM2JmZmFkY2U5NDNkOGU3MDJhZDE0YTk2OTY2NjQ0NjYuanBn-.jpg",
"cloth_type": "upper_body",
"file_prefix": "284c4f3d-4bf6-4bad-a603-d2a1948de0cf",
"guidance_scale": 7.5,
"image": "https://pub-3626123a908346a7a8be8d9295f44e26.r2.dev/livewire-tmp/5dzoZ9qWI2FQxwceFDb3zULRtwCRmF-metaZjA5NjMyX3BhcmVudF8xXzE2NTMwMDMzODguanBn-.jpg",
"negative_prompt": "low quality",
"num_inference_steps": 20,
"num_samples": 1,
"outdir": "out",
"prompt": ":A realistic photo of a model wearing a beautiful t-shirt",
"refine_face": "yes",
"scale": 5,
"seed": 970128919,
"temp": "no",
}
}